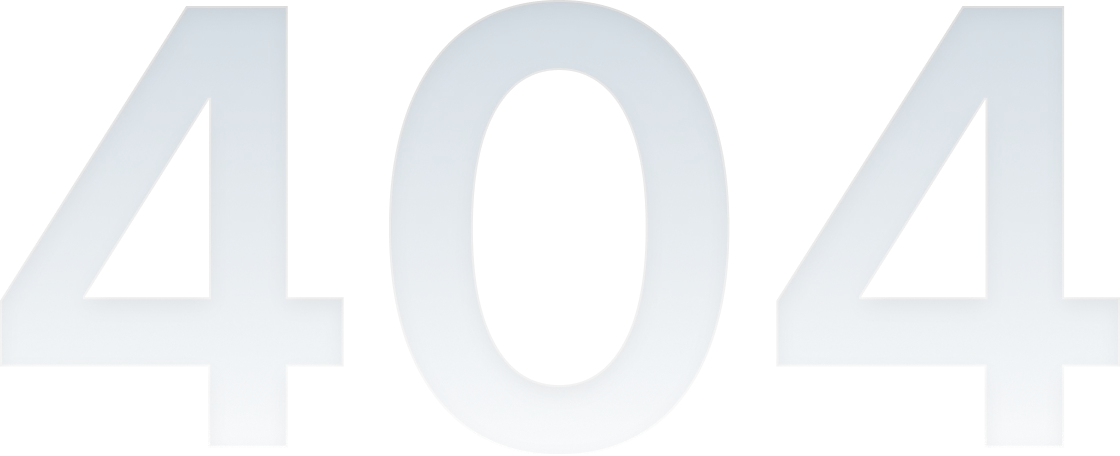
Page not found
We’re sorry, but we can’t find the page you were looking for. It’s probably some thing we’ve done wrong but now we know about it and we’ll try to fix it.
We’re sorry, but we can’t find the page you were looking for. It’s probably some thing we’ve done wrong but now we know about it and we’ll try to fix it.